Tutorial 01 - 3DS Max HLSL Shader Basics |
|
This tutorial will explain how to make a basic shader with a color control for 3DS Max. |
|
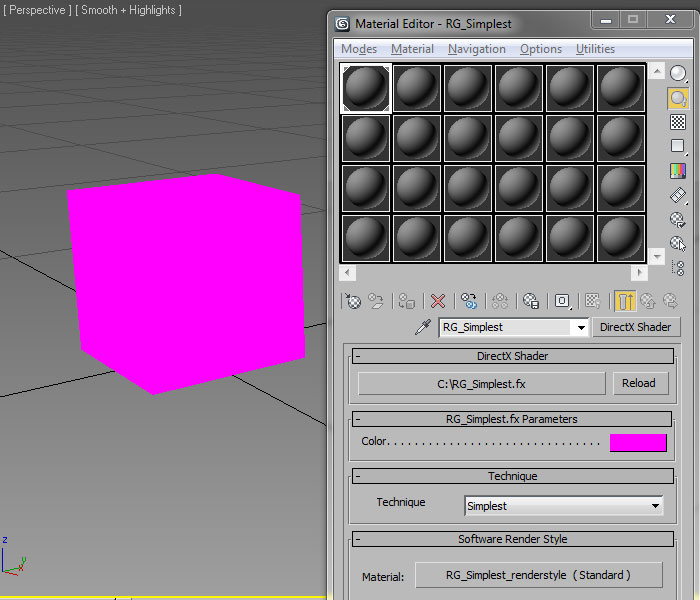
|
|
|
Sample Code:
|
First, lets look at the completed shader: |
|
• • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • |
// HLSL Simple Shader for 3DS Max
// Ruben Garza
// http://www.rubengarza.com/shader-T01-Basics.htm
// Global Variables
float4x4 mvpMatrix : WorldViewProj; // World View Model*View*Projection
// 3DS Max UI Elements
float4 Color
<
string UIName = "Color"; // Name Displayed in material editor
float UIMin = 0.0f; // Minimum spinner value
float UIMax = 1.0f; // Maximum spinner value
> = float4( 1.0f, 0.00f, 1.00f, 1.0f ); // Default diffuse color
// Vertex Shader
struct vertShader // Struct contains output variables
{
float4 Position : POSITION; // position in projection space
};
vertShader SimpleVS // List of variables passed in
(
float4 vertPos : POSITION, // vertex position in object space
uniform float4x4 mvpMx // mvpMatrix from global
)
{ // Runs commands below on every vertex
vertShader OUT; // output position from vertShader struct
OUT.Position = mul(vertPos,mvpMx).xyzw; // transform position to projection space
return OUT; // Outputs the variables listed in struct
}
// Pixel Shader
float4 SimplePS(vertShader IN): COLOR // Inputs variable from struct
{ // Runs commands below on every pixel
pixelShader OUT; // output color from pixelShader struct
return Color; // Outputs color from the material editor
}
// Technique
technique Simplest // Name of technique
{
pass p0
{
ZEnable = true;
ZWriteEnable = true;
CullMode = None;
VertexShader = compile vs_1_1 SimpleVS(mvpMatrix); // Compile vertex shader
PixelShader = compile ps_2_0 SimplePS(); // Compile pixel shader
}
} |
• • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • |
|
HLSL shaders usually consist of several components:
- Global variables and functions
- 3DS Max Specific shader controls
- Vertex Shader
- Pixel Shader
- Technique
|
|
Globals: |
Globals variables are used by the pixel and vertex shaders to display the model correctly. |
In this example we have the world view projection matrix that is passed into the shader from 3ds Max. |
This is the transform matrix from model space to clip space which allows the model to be rendered to the screen. |
|
3DS Max Controls: |
Spinners, color pickers, and other UI controls can be defined in the shader. These controls will be exposed in the material editor. |
|
float4 Color
<
string UIName = "Color"; // Name Displayed in material editor
float UIMin = 0.0f; // Minimum spinner value
float UIMax = 1.0f; // Maximum spinner value
> = float4( 1.0f, 0.00f, 1.00f, 1.0f ); // Default diffuse color |
|
float4 means a variable with 4 values, in this case r,b,g, and alpha.
The string UIName is what is displayed in the material editor.
The UIMin and UIMax set the limits on the values.
The float4 in the last line is the default value, in this case magenta. |
|
Vertex Shader: |
The vertex shader is a function that is run on every vertex. |
|
The vertex shader uses a container called a struct (short for structure) to define what it outputs to the pixel shader. |
struct vertShader // Struct contains output variables
{
float4 Position : POSITION; // position in projection space
}; |
In this case the struct just outputs the vertex positions to the pixel shader. |
|
TheSimpleVS function does the work of processing the verts. |
vertShader SimpleVS // List of variables passed in
(
float4 vertPos : POSITION, // vertex position in object space
uniform float4x4 mvpMx // mvpMatrix from global
)
{ // Runs commands below on every vertex
vertShader OUT; // output position from vertShader struct
OUT.Position = mul(vertPos,mvpMx).xyzw; // transform position to projection space
return OUT; // Outputs the variables listed in struct
}; |
The function outputs each vertex position in projection space so that it can be rendered to the screen.
The vertShader at the beginning tells the function what it will output - in this case just a float4 for the position. |
|
Pixel Shader: |
The pixel shader is a program that is run on every pixel of the object on every frame. |
|
Technique: |
Techniques are what tie all the elements together. |
|
|
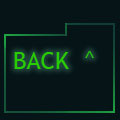
|